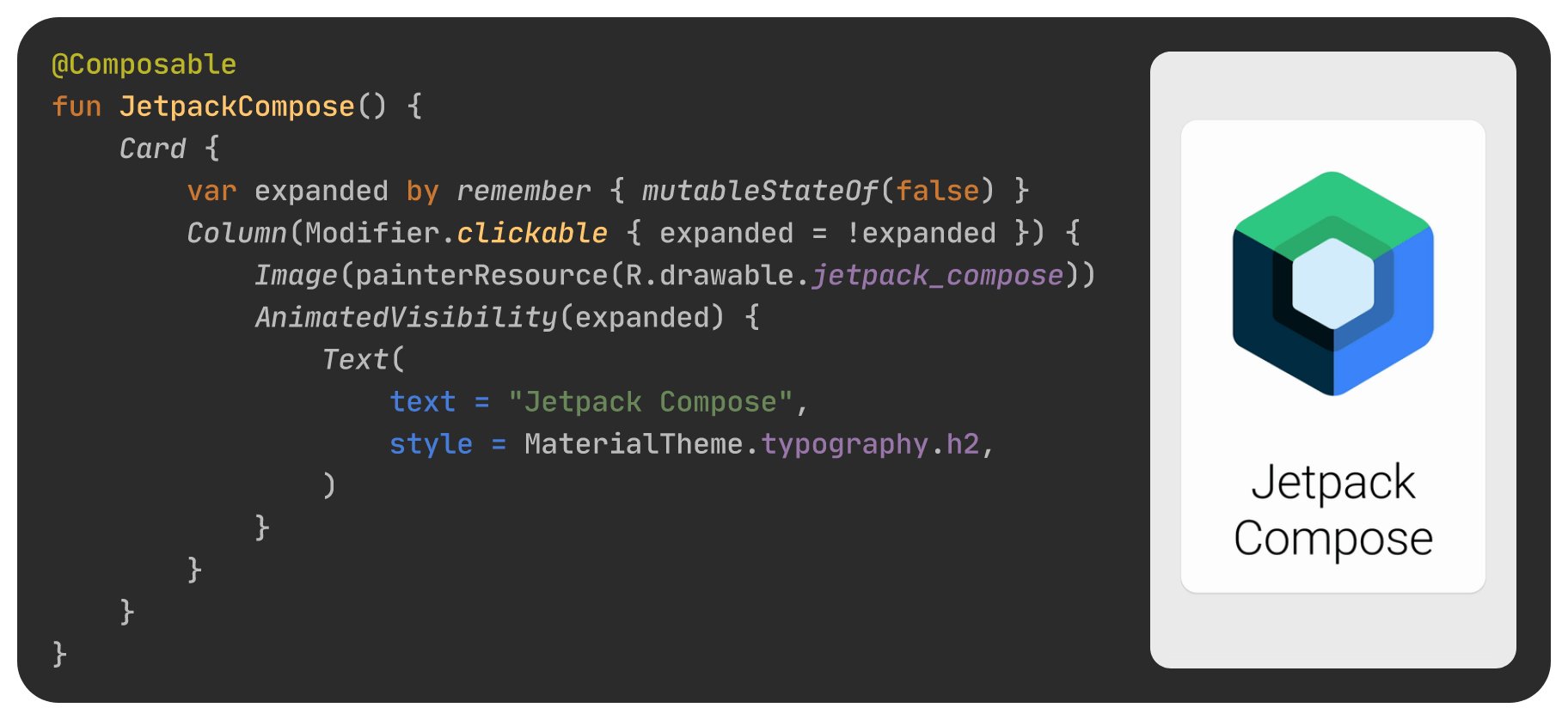
Overview
Andoid Compose에서 Animation을 지원하기 위한 Composable 이나 State 에 대해서 정리한 포스트입니다.
1. animateColorAsState()
Color 값을 변경했을 때 이를 자연스럽게 표현하기 위한 State입니다.
val backgroundColor = if (tabPage == TabPage.Home) Purple100 else Green300
Scaffold(
...
backgroundColor = backgroundColor,
...
)
위 코드를 적용하였을 때 Scaffold의 Color가 변화는 과정을 애니메이션으로 표현하게 됩니다.

2. AnimatedVisibility()
Composable를 보여지고 사라지는 과정을 애니메이션으로 표현해주는 Composable 입니다.
var extended : Boolean
...
AnimatedVisibility(extended) {
Text(
text = stringResource(R.string.edit),
modifier = Modifier
.padding(start = 8.dp, top = 3.dp)
)
}
AnimatedVisibility 에 지정된 Boolean값이 변경 될 때마다 애니메이션을 실행합니다.
기본적으로 AnimatedVisibility 의 자식 Composable을 페이드 인 및 확장하여 표시하고 페이드 아웃 및 축소하여 숨깁니다.

AnimatedVisibility 에 enter
와 exit
매개 변수를 사용하여 Composable이 보여지고 사라지는 사용자 정의 애니메이션을를 사용할 수 있습니다.
AnimatedVisibility(
visible = shown,
enter = slideInVertically(
// Enters by sliding down from offset -fullHeight to 0.
initialOffsetY = { fullHeight -> -fullHeight },
animationSpec = tween(durationMillis = 150, easing = LinearOutSlowInEasing)
),
exit = slideOutVertically(
// Exits by sliding up from offset 0 to -fullHeight.
targetOffsetY = { fullHeight -> -fullHeight },
animationSpec = tween(durationMillis = 250, easing = FastOutLinearInEasing)
)
) {
...
}
enter
매개변수에 EnterTransition
을 인스턴스화 하여 넘겨주며 EnterTransition
는 해당 Composable의 시작 위치 및 AnimationSpec
를 정의합니다.
그리고 exit 매개변수에 ExitTransition
을 인스턴스화 하여 넘겨주며 ExitTransition
는 해당 Composable의 종료 위치 및 AnimationSpec
를 정의합니다.
위 매개변수를 통해 Composable이 위/아래 방향으로 보여지고 사라지는 것을 볼수 있습니다.

AnimatedVisibility의 디폴트 매개변수는 아래와 같습니다.
@Composable
fun AnimatedVisibility(
visible: Boolean,
modifier: Modifier = Modifier,
enter: EnterTransition = fadeIn() + expandIn(),
exit: ExitTransition = shrinkOut() + fadeOut(),
content: @Composable() AnimatedVisibilityScope.() -> Unit
) {
...
}
3. Modifier.animateContentSize()
Composable 함수 내부에 사이즈가 변경이 되거나 컨텐츠가 변경이 될 경우 동적으로 사이즈 변화를 애니메이션으로 처리가 됩니다.
Column(
modifier = Modifier
.fillMaxWidth()
.padding(16.dp)
.animateContentSize()
) {
// Column 안의 내용물이 변경이 된 경우
}

4. InfiniteTransition
영구적으로 애니메이션을 처리하기 위한 객체입니다.
val alpha by infinityTransition.animateFloat(
initialValue = 0.0f, targetValue = 1f, animationSpec = infiniteRepeatable(
animation = keyframes {
durationMillis = 1000
0.7f at 500
},
repeatMode = RepeatMode.Reverse
)
)
위 코드는 infinityTransition 델리게이트를 통해 alpha 값이 0 .. 1 .. 0 으로 계속 변하게 되며
위 alpha 값을 Composable의 alpha 매개변수에 set을 하게되면
Box(
modifier = Modifier
.size(48.dp)
.clip(CircleShape)
.background(Color.LightGray.copy(alpha = alpha))
)
아래와 같이 반짝이는 애니매이션 효과를 나타내게 됩니다.

댓글남기기